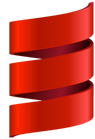
Scala is a general purpose programming language that compiles to Java bytecode, therefore, it will run on the
JVM
. You can code is Scala using an object-oriented approach or a functional approach but almost everybody who codes in Scala tries to do it using the second one. But, why?
You only need to search on Google and you will find the benefits that are told for many people about immutability like: Thread Safe, Concurrency, Scalability, Maintainability, Performance and many more.
This post will not try to explain these benefits or even to convince you about the immutability. It will only try to perform a very easy coding test. I will try to solve a
project-euler
's problem using both approaches, specifically, the
problem 2
.
Even Fibonacci numbers
Each new term in the Fibonacci sequence is generated by adding the previous two terms. By starting with 1 and 2, the first 10 terms will be:
1, 2, 3, 5, 8, 13, 21, 34, 55, 89, ...
By considering the terms in the Fibonacci sequence whose values do not exceed four million, find the sum of the even-valued terms.
Code
:
object Problem2 { def main(args: Array[String]): Unit = { println("Project Euler 2\n") val number: Int = 4000000 println("Mutability Result: \t" + time({mutable(number)}, "Mutability Time: \t")) println() println("Immutability Result: \t" + time({immutable(number)}, "Immutability Result: \t")) } def time[R](block: => R, introMessage:String): R = { val t0 = System.nanoTime() val result = block val t1 = System.nanoTime() println(introMessage + (t1 - t0) + "ns") result } def mutable(number: Int): Int = { var (first, second, acc) = (1, 2, 2) while (second < number) { val aux = second second += first first = aux if (second % 2 == 0) acc += second } acc } def immutable(number: Int): Int = { immutableFib(1, 2, number, 2) } def immutableFib(first: Int, second: Int, number: Int, acc: Int): Int = { second match { case _ if second < number => val next = first + second val newAcc = if (next % 2 == 0) acc + next else acc immutableFib(second, next, number, newAcc) case _ => acc } } }
Results:
Project Euler 2 Mutability Time: 1506573ns Mutability Result: 4613732 Immutability Time: 25331ns Immutability Result: 4613732
Note: Without the time wrapper, measuring directly the elapsed time, the results are much better.
Although the two algorithms obtain the same result (
All roads lead to Rome
) you can see which kind of code will be more maintainable, will work better when you build concurrent systems and will perform better.
It is true that coding in a functional way is not easy, especially when the language has not been designed to do it, but in Scala you can choose the way, therefore, it is up to you.